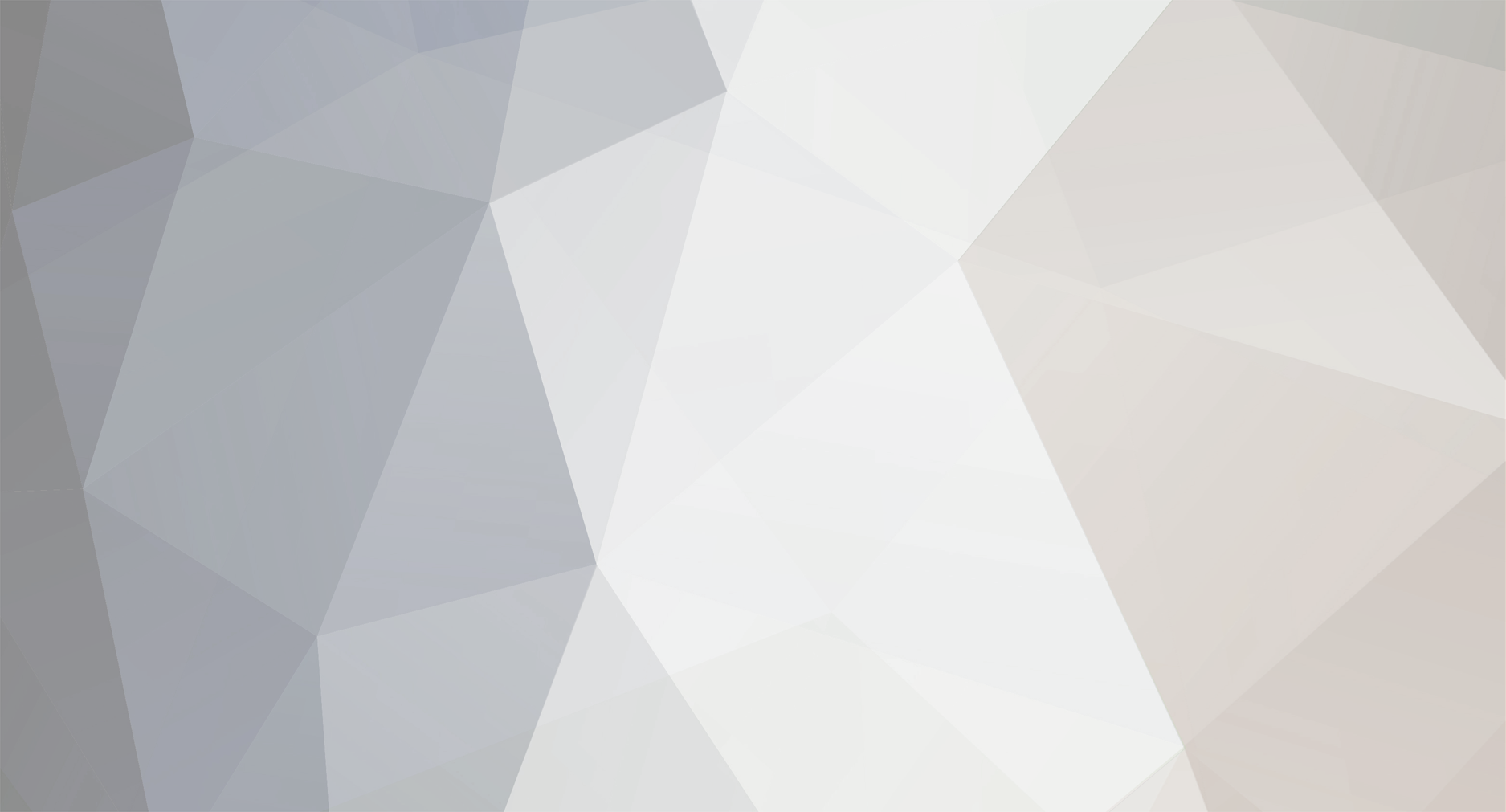
Happy Secret
-
Posts
539 -
Joined
-
Last visited
Posts posted by Happy Secret
-
-
1 hour ago, Gay 228 said:
the field I need is in the CCDrivencontroller
do i need a config??Then you don’t need.
Can you try if you can cheat with option 2 first?
-
48 minutes ago, Gay 228 said:
I need to change the height of the jump
yes i can get everything from ccdrivencontroller
this game: Oxide survival island (survival)
what about _config, I don't quite understand what it is responsible for in the code, can you explain?Can you cheat this high jump with H5GG enhanced menu alone?
if the only class object you need is ccdrivencontroller. Then you don’t need to travel to other class object.
_config is just an example that I need to travel from CharacterMotor object to CharacterMotorConfig object
-
3 minutes ago, Gay 228 said:
/*
H5GG Plugin Mod Menu logic should enable easier Mod Menu development for non-jailbroken. It preserve existing H5GG UI and features, while adding new cheat menu.
Cheat implemented using a patch free approach, the game binary does not required to patch.
Contribute by Happy Secret on iOSGods (2023)
*/
var script = initializeUnitySupport();//[MODIFY] Configure Cheat Here
var cheats = {
JumpHeight: ["CCDrivenController", "m_JumpHeight", "50", "SELF", "ON"],
};var intervalID;
var aryObj
var cheatState = false;
var cheatMode = "recurrent";
var recurrentInterval = 1200; //try not to be small than 1000, prevent system freezefunction toogleCheat() {
cheatState = !cheatState;
document.getElementById("applybutton").textContent = ' Toggle OFF ';
document.getElementById("applybutton").style.backgroundColor = '#E8E8E850';if (cheatState) {
intervalID = setInterval(function () {
if (cheatState) {
try {
script = initializeUnitySupport();
//[MODIFY]Find core object object list
aryObj = script.call("findUnityObjectOfType", ["Oxide$CCDrivenController", true]);
} catch (e) {
//reset Unity Support
gIl2cppInit = false;
var script = initializeUnitySupport();
appendLog("Unity support crashed and reset complete");
}if (!aryObj) {
appendLog("Cannot find object to cheat. Engine stopped.");
cheatState = false;
document.getElementById("applybutton").textContent = ' Toggle Cheat ';
return;
} else if (aryObj.length == 0) {
appendLog("Cheat waiting for core object.");
return;
}
for (let i = 0; i < aryObj.length; i++) {
//[MODIFY]Start prepare core object for delink / filtering here
let CharacterMotor = new UnityObject(aryObj[i]);
//debugInfo("BattleCharacter (" + aryObj[i].toString(16) + ") with UnityObject:" + BattleCharacter, [aryObj[i]])
CharacterMotor.loadFields(["_config"]);
//debugInfo("BattleCharacter (" + aryObj[i].toString(16) + ") with player:" + BattleCharacter.Status, [aryObj[i]])
//debugInfo("BattleCharacter (0x" + aryObj[i].toString(16) + ") with CurrentUnitType:" + BattleCharacter.CurrentUnitType, [aryObj[i]])
//[MODIFY]Locate the right object to cheat (SELF)
let isSelf = true//BattleCharacter.CurrentUnitType==100? true:false;
let cheatObj = {};
//End prepare core object for delink / filtering here
//[MODIFY]Start prepare cheat object here
let CharacterMotorConfig = new UnityObject(CharacterMotor._config)
CharacterMotorConfig.loadFields(["m_JumpHeight"])
cheatObj["CCDrivenController"] = CharacterMotorConfig
//End prepare cheat object
for (var prop in cheats) {
if (Object.prototype.hasOwnProperty.call(cheats, prop)) {
let obj;
let addr;
let oldValue = 0;
if (isNaN(cheats[prop][2])){
switch (cheats[prop][2]){
case "ONE":
cheats[prop][2] = 1
break;
}
}
if (isSelf && cheats[prop][3] == "SELF" && cheats[prop][4] == "ON") {
cheatObj[cheats[prop][0]][cheats[prop][1]] = isNaN(cheats[prop][2]) ? cheatObj[cheats[prop][0]][cheats[prop][2]] : cheats[prop][2];
} else if (isSelf==false && cheats[prop][3] == "ENEMY" && cheats[prop][4] == "ON") {
cheatObj[cheats[prop][0]][cheats[prop][1]] = isNaN(cheats[prop][2]) ? cheatObj[cheats[prop][0]][cheats[prop][2]] : cheats[prop][2];
}//end if TURN ON
}
}//end For cheat item
}//end For core object list
appendLog("Cheat applied successfully");
} else {
clearInterval(intervalID);
}
}, recurrentInterval);
} else {
//code will stop on next coming iteration
document.getElementById("applybutton").textContent = ' Toggle Cheat ';
appendLog("Cheat stopped");
}if (cheatMode != "recurrent") clearInterval(intervalID);
}/* Add button on H5GG UI to open the Cheat UI, if it does not exist */
if ($("#cheatpluginmodmenu").length == 0) {
var btn = $('<button id="cheatpluginmodmenu">Cheat</button>');
btn.attr("credit", "Happy Secret");
btn.click(function () {
$("#cheatpluginpage").show();
});
//backward compatible with standard H5GG menu
if ($("#frontview").length == 0) {
$('#results_count').after(btn);
} else {
$("#frontview").append(btn);
}
}/* Create the Cheat UI Layer, if it does not exist */
if ($("#cheatpluginpage").length == 0) {
var popup_pixelfancheat_html = $('<div id="cheatpluginpage" style="background-color: #FDFDFD; width:100%; height:100%; position:absolute; left:0; top:0; border:1px solid #B8B8B880; border-radius: 5px; padding: 0px; z-index: 99999;-webkit-user-select: all;-webkit-touch-callout: default;"></div>');
$(document.body).append(popup_pixelfancheat_html);
}/* Generate Clean Cheat Menu UI */
var html = ' Cheat by Happy Secret';
html += '<br><div style="float:right; font-size:16px; font-family: Arial, sans-serif;" onclick="closecheatpluginpage()"> X </div>';/* Prepare Cheat Item */
for (var prop in cheats) {
if (Object.prototype.hasOwnProperty.call(cheats, prop)) {
if (isNaN(cheats[prop][2]))
html += '<label><input name="' + prop + '"type="checkbox" ' + '" onchange="checkchange(this)" checked/>' + prop + '<output>(' + cheats[prop][4] + ')</output></label>';
else
html += '<br><label><input name="' + prop + '"type="range" min="0" max="' + cheats[prop][2] * 2 + '" value="' + cheats[prop][2] + '" style="width:50%" onchange="rangechange(this)" />' + prop + '<output>(' + cheats[prop][2] + ')</output></label>';
}
}html += '<p align="center"><button onclick="toogleCheat()" id="applybutton"> Toggle Cheat </button>';
html += '</hr><div id="cheatpluginpagelog" class="scrollbar" ></div>';
$("#cheatpluginpage").html(html);
$("#cheatpluginpage").hide();function closecheatpluginpage() {
$("#cheatpluginpage").hide();
}function rangechange(input) {
cheats[input.name][2] = Number(input.value);input.nextElementSibling.value = '(' + input.value + ')';
//input.previousElementSibling.value = '(' + input.value + ')';//Change Apply Button Color to remind user to press Apply
document.getElementById("applybutton").style.backgroundColor = 'yellow';
}function checkchange(input) {
cheats[input.name][4] = input.checked ? "ON" : "OFF"input.nextElementSibling.value = '(' + cheats[input.name][4] + ')';
//Change Apply Button Color to remind user to press Apply
document.getElementById("applybutton").style.backgroundColor = 'yellow';
}/* Create Cheat Log */
function appendLog(msg) {
var oldmsg = $("#cheatpluginpagelog").html();
if (oldmsg.length > 300) oldmsg = "";
oldmsg = getCurTime() + ' - ' + msg + '<br>' + oldmsg;
$("#cheatpluginpagelog").html(oldmsg);
}yes, of course, the script is in the spoiler
If you want to test out Unity User Object wrapper, I suggest you to use Option 2 first.
from you script, I have few questions.
1. Are you sure you can get object pointer for this class Oxide$CCDrivenController
2. Your step 3, doesn’t look right. You are also using _config??CharacterMotor.loadFields(["_config"]);
3. Your step 5, doesn’t look right as well.
let CharacterMotorConfig = new UnityObject(CharacterMotor._config)???What game is it?
If you are using you can get an object from Oxide$CCDrivenController. And you just need to update m_JumpHeight from this object.
Your step 3 should be
CharacterMotor.loadFields(["m_JumpHeight"]); /l
You may simplify step 5, to just put CCDrivenController object to cheatObj["CCDrivenController"] = CharacterMotor (or you should rename it)
-
2
-
-
4 hours ago, pony945 said:
I found out the "_hp" have been Secured.
So I change the onHitKill _hp to maxHP and off the hp/mp hack.
It work, but I'm not sure if it's safe.
Issue resolved. Please download again.
-
1
-
-
1 hour ago, Gay 228 said:
I did everything like normal, the name of the object is CCDrivenController and the name of the field is m_JumpHeight
not work(Can you share your few lines of code to wrap the object and load the field? Or simply share the entire script
-
1 hour ago, pony945 said:
I found out the "_hp" have been Secured.
So I change the onHitKill _hp to maxHP and off the hp/mp hack.
It work, but I'm not sure if it's safe.
Thanks for the update.
Yes, Game Developer made changes on HP and MP.
max HP seems the temporary thing that we can use for now. But I guess game developer will fix this in next release.
let me think about it
-
1
-
-
30 minutes ago, XXOOXXA said:
Can i know where I can find it ? I’m Willing to learn
Inside the top post, inside the second Reveal Hidden Content.
-
1 hour ago, XXOOXXA said:
Hi @Happy Secret can update to new version. Thanks ☺️
This game has weekly update schedule.
I strongly suggest you learn to do DIY by following the instructions I provided.This give you shortest time to get the cheat apply on new version
-
7 hours ago, Gay 228 said:
is it possible to run in h5gg to test the feature?
Not sure what you mean. Of course the script need to run with H5GG
-
4 hours ago, Gay 228 said:
This normally happens when you have wrong object pointer, class name, or field name.
-
While people start cheating with H5GG Enhanced Menu on Unity Game, they might then looking for way to execute the cheat in a convenient way.
JSPlug-in is obviously the ANSWER to this.
JSPlug-in is a framework setup of H5GG Enhanced Menu which allow you to extend the H5GG menu with new features, such as cheat menu for a game.
Under this Framework setup, user can leverage prebuilt H5GG Enhanced Menu function in their own user cheat. This largely simplify the complexity of the script, while having amazing features in it. To name a few:
1. Direct retrieve of Unity object pointers (Covering on Scene User Object, Singleton, any object that could link from object already found)
2. Use Unity dump structure to define the cheat without the need to hard code any offset. This essentially relieve people's headache in preparing cheat updates for game updates, because there is no more script update required.
3. Javascript as base, allow easy create cheat menuWith latest release of H5GG Enhanced Menu v1.8.4 onwards, writing JSPlug-in is getting easier. The introduction of Unity User Object wrapper, seamlessly expose Unity User Object to Javascript.
- User can access to Unity User Object's Fields and Methods directly from Javascript. Get / Set / Call and return, everything becomes so initiative.Here let's go through a quick example to demonstrate how powerful yet easy, JSPlug-in is.
Game: Subway Surfers (Any version)
Cheat feature: Big Jump (jump up very high)For those who want to know how to use H5GG Enhanced Menu to cheat this. You may see below tutorial from @impapas.
SpoilerThere are multiple way to implement this with JSPlug-in, it is all available for you to choose from.
Option 1) Minimum number of lines of code. 3 lines only.
Spoilervar script = initializeUnitySupport(); var aryObj = script.call("findUnityObjectOfType", ["SYBO$RunnerCore$Character$CharacterMotor", true]); writeFloat(readPtr(Number(aryObj[0])+Number(gUnityClasses.SYBO$RunnerCore$Character$CharacterMotor._config.offset))+Number(gUnityClasses.SYBO$RunnerCore$Character$CharacterMotorConfig.JumpHeight.offset),50)
NOTE:
1. Line 1 initialise the Unity Support in JSPlug-in
2. Line 2 retrieve an array of Unity User Object of type - SYBO$RunnerCore$Character$CharacterMotor. This is the naming convention used in H5GG Enhanced Menu for Class. It essentially replace the dot(.) of Unity namespace and class name into dollar sign($).
e.g. SYBO.RunnerCore.Character.CharacterMotor -> SYBO$RunnerCore$Character$CharacterMotor
This CharacterMotor class is a class that we can easily retrieve its runtime object pointer and has relation with the CharacterMotorConfig class that holds the data we want to cheat
3. Line 3 retrieve CharacterMotorConfig object pointer from CharacterMotor's _config field, then write new value to CharacterMotorConfig's JumpHeight fieldOption 2) Easiest to understand. 7 lines (4 lines more) but more readable.
Spoilervar script = initializeUnitySupport(); var aryObj = script.call("findUnityObjectOfType", ["SYBO$RunnerCore$Character$CharacterMotor", true]); var CharacterMotor = new UnityObject(aryObj[0]) CharacterMotor.loadFields(['_config']) var CharacterMotorConfig = new UnityObject(CharacterMotor._config) CharacterMotorConfig.loadFields(['JumpHeight']) CharacterMotorConfig.JumpHeight = 50
NOTE:
1. Line 1 and 2, are the same as Option 1
2. Line 3 uses Unity User Object Wrapper (UnityObject) to wrap CharacterMotor's object pointer
3. Line 4 tell JSPlug-in to load _config field's meta data and enable direct access
4. Line 5 uses Unity User Object Wrapper (Unity Object) to wrap CharacterMotorConfig's object pointer retrieved from CharacterMotor._config
YES, dot(.) notation, you can use [] notation as well - CharacterMotor["_config"]
5. Line 6 tell JSPlug-in to load JumpHeight field's meta data and enable direct access
6. Line 7 write new value to CharacterMotorConfig.JumpHeight directly
YES, dot(.) notation, you can use [] notation as well - CharacterMotorConfig["JumpHeight"] = 50Option 3) Wrap everything with a Cheat Menu.
Spoiler/* H5GG Plugin Mod Menu logic should enable easier Mod Menu development for non-jailbroken. It preserve existing H5GG UI and features, while adding new cheat menu. Cheat implemented using a patch free approach, the game binary does not required to patch. Contribute by Happy Secret on iOSGods (2023) */ var script = initializeUnitySupport(); //[MODIFY] Configure Cheat Here var cheats = { JumpHeight: ["CharacterMotorConfig", "JumpHeight", "50", "SELF", "ON"], }; var intervalID; var aryObj var cheatState = false; var cheatMode = "recurrent"; var recurrentInterval = 1200; //try not to be small than 1000, prevent system freeze function toogleCheat() { cheatState = !cheatState; document.getElementById("applybutton").textContent = ' Toggle OFF '; document.getElementById("applybutton").style.backgroundColor = '#E8E8E850'; if (cheatState) { intervalID = setInterval(function () { if (cheatState) { try { script = initializeUnitySupport(); //[MODIFY]Find core object object list aryObj = script.call("findUnityObjectOfType", ["SYBO$RunnerCore$Character$CharacterMotor", true]); } catch (e) { //reset Unity Support gIl2cppInit = false; var script = initializeUnitySupport(); appendLog("Unity support crashed and reset complete"); } if (!aryObj) { appendLog("Cannot find object to cheat. Engine stopped."); cheatState = false; document.getElementById("applybutton").textContent = ' Toggle Cheat '; return; } else if (aryObj.length == 0) { appendLog("Cheat waiting for core object."); return; } for (let i = 0; i < aryObj.length; i++) { //[MODIFY]Start prepare core object for delink / filtering here let CharacterMotor = new UnityObject(aryObj[i]); //debugInfo("BattleCharacter (" + aryObj[i].toString(16) + ") with UnityObject:" + BattleCharacter, [aryObj[i]]) CharacterMotor.loadFields(["_config"]); //debugInfo("BattleCharacter (" + aryObj[i].toString(16) + ") with player:" + BattleCharacter.Status, [aryObj[i]]) //debugInfo("BattleCharacter (0x" + aryObj[i].toString(16) + ") with CurrentUnitType:" + BattleCharacter.CurrentUnitType, [aryObj[i]]) //[MODIFY]Locate the right object to cheat (SELF) let isSelf = true//BattleCharacter.CurrentUnitType==100? true:false; let cheatObj = {}; //End prepare core object for delink / filtering here //[MODIFY]Start prepare cheat object here let CharacterMotorConfig = new UnityObject(CharacterMotor._config) CharacterMotorConfig.loadFields(["JumpHeight"]) cheatObj["CharacterMotorConfig"] = CharacterMotorConfig //End prepare cheat object for (var prop in cheats) { if (Object.prototype.hasOwnProperty.call(cheats, prop)) { let obj; let addr; let oldValue = 0; if (isNaN(cheats[prop][2])){ switch (cheats[prop][2]){ case "ONE": cheats[prop][2] = 1 break; } } if (isSelf && cheats[prop][3] == "SELF" && cheats[prop][4] == "ON") { cheatObj[cheats[prop][0]][cheats[prop][1]] = isNaN(cheats[prop][2]) ? cheatObj[cheats[prop][0]][cheats[prop][2]] : cheats[prop][2]; } else if (isSelf==false && cheats[prop][3] == "ENEMY" && cheats[prop][4] == "ON") { cheatObj[cheats[prop][0]][cheats[prop][1]] = isNaN(cheats[prop][2]) ? cheatObj[cheats[prop][0]][cheats[prop][2]] : cheats[prop][2]; }//end if TURN ON } }//end For cheat item }//end For core object list appendLog("Cheat applied successfully"); } else { clearInterval(intervalID); } }, recurrentInterval); } else { //code will stop on next coming iteration document.getElementById("applybutton").textContent = ' Toggle Cheat '; appendLog("Cheat stopped"); } if (cheatMode != "recurrent") clearInterval(intervalID); } /* Add button on H5GG UI to open the Cheat UI, if it does not exist */ if ($("#cheatpluginmodmenu").length == 0) { var btn = $('<button id="cheatpluginmodmenu">Cheat</button>'); btn.attr("credit", "Happy Secret"); btn.click(function () { $("#cheatpluginpage").show(); }); //backward compatible with standard H5GG menu if ($("#frontview").length == 0) { $('#results_count').after(btn); } else { $("#frontview").append(btn); } } /* Create the Cheat UI Layer, if it does not exist */ if ($("#cheatpluginpage").length == 0) { var popup_pixelfancheat_html = $('<div id="cheatpluginpage" style="background-color: #FDFDFD; width:100%; height:100%; position:absolute; left:0; top:0; border:1px solid #B8B8B880; border-radius: 5px; padding: 0px; z-index: 99999;-webkit-user-select: all;-webkit-touch-callout: default;"></div>'); $(document.body).append(popup_pixelfancheat_html); } /* Generate Clean Cheat Menu UI */ var html = ' Cheat by Happy Secret'; html += '<br><div style="float:right; font-size:16px; font-family: Arial, sans-serif;" onclick="closecheatpluginpage()"> X </div>'; /* Prepare Cheat Item */ for (var prop in cheats) { if (Object.prototype.hasOwnProperty.call(cheats, prop)) { if (isNaN(cheats[prop][2])) html += '<label><input name="' + prop + '"type="checkbox" ' + '" onchange="checkchange(this)" checked/>' + prop + '<output>(' + cheats[prop][4] + ')</output></label>'; else html += '<br><label><input name="' + prop + '"type="range" min="0" max="' + cheats[prop][2] * 2 + '" value="' + cheats[prop][2] + '" style="width:50%" onchange="rangechange(this)" />' + prop + '<output>(' + cheats[prop][2] + ')</output></label>'; } } html += '<p align="center"><button onclick="toogleCheat()" id="applybutton"> Toggle Cheat </button>'; html += '</hr><div id="cheatpluginpagelog" class="scrollbar" ></div>'; $("#cheatpluginpage").html(html); $("#cheatpluginpage").hide(); function closecheatpluginpage() { $("#cheatpluginpage").hide(); } function rangechange(input) { cheats[input.name][2] = Number(input.value); input.nextElementSibling.value = '(' + input.value + ')'; //input.previousElementSibling.value = '(' + input.value + ')'; //Change Apply Button Color to remind user to press Apply document.getElementById("applybutton").style.backgroundColor = 'yellow'; } function checkchange(input) { cheats[input.name][4] = input.checked ? "ON" : "OFF" input.nextElementSibling.value = '(' + cheats[input.name][4] + ')'; //Change Apply Button Color to remind user to press Apply document.getElementById("applybutton").style.backgroundColor = 'yellow'; } /* Create Cheat Log */ function appendLog(msg) { var oldmsg = $("#cheatpluginpagelog").html(); if (oldmsg.length > 300) oldmsg = ""; oldmsg = getCurTime() + ' - ' + msg + '<br>' + oldmsg; $("#cheatpluginpagelog").html(oldmsg); }
NOTE:
1. This is a simple cheat menu template. It will automatically create Slider or Checkbox for cheats. It automatically run the cheat every 1200 milliseconds.
2. You do not need to understand the entire menu code in order to use it. There essentially 5 Steps to use this template script
STEP 1) Define what you want to cheat with the script
//[MODIFY] Configure Cheat Here var cheats = { JumpHeight: ["CharacterMotorConfig", "JumpHeight", "50", "SELF", "ON"], };
- First JumpHeight is the label of the slider
- CharacterMotorConfig is the name of the class that hold the field you want to change
- Second JumpHeight is the field name to change
- 50 is the value to change. Use "ONE" for cheat that only need to enable/disable without a numeral value.
- SELF is for de-link, it used to tell which group of object should apply this cheat on (Filtering condition)
- ON is a switch to turn on and off the cheatSTEP 2) Change the root object of interest, which should able to link to your other cheat object
//[MODIFY]Find core object object list aryObj = script.call("findUnityObjectOfType", ["SYBO$RunnerCore$Character$CharacterMotor", true]);
Change the “SYBO$RunnerCore$Character$CharacterMotor” to an object class that you can find object link with Unity Static Analyzer. You need to full long name with those $.
STEP 3) Create the Unity User Object wrapper object from a Root Object instance address, and enable the linking field access
//[MODIFY]Start prepare core object for delink / filtering here let CharacterMotor = new UnityObject(aryObj[i]); CharacterMotor.loadFields(["_config"]);
Here create the base Unity Object, you just need to name it and identify that Field In this object you are going to use
STEP 4) Define the scope of the cheat, certain cheat we do not want to globally apply. (Filtering condition or Grouping)
//[MODIFY]Locate the right object to cheat (SELF) let isSelf = true; //BattleCharacter.CurrentUnitType==100? true:false;
This is used to delink. Delink mean, cheat apply to certain object not all. Say, only on myself but not enemy. We want to amplify our team's attack but not enemy's team.
STEP 5) Create the Unity User Object wrapper object from a Root Object instance address, and enable the linking field access
//[MODIFY]Start prepare cheat object here let CharacterMotorConfig = new UnityObject(CharacterMotor._config) CharacterMotorConfig.loadFields(["JumpHeight"]) cheatObj["CharacterMotorConfig"] = CharacterMotorConfig
Like the earlier step, you setup the cheat Unity Object here and put it under the cheatObj
If you have 3 different Unity User Objects, which holds 5 fields you want to cheat. Here, you will have 3 set of above logics with each defines one of the Unity User Object.
Just to remember, EVERY SINGLE field we use, we need to load them explicitly.With this 5 Steps, you should able to create simple Cheat Menu for Simple Unity Games.
This is obviously not for all Unity Games. Some Unity Games can't be cheated without Patching / Hooking.
New Badge
1. As they are JSPlug-in, in order to enable autoload on these script when H5GG startup and trigger. You need to define the name with prefix "H5JSPlugin - " with extension ".js".
e.g. H5JSPlugin - Subway Surfers.js
2. And you will need to put this Javascript file either in Document folder or .app folder (app bundle).Please read Release note of H5GG Enhanced Menu for detail
-
28
-
2
-
1
-
1
-
3
-
4
-
-
54 minutes ago, Selvam g said:
Cài như nào bạn hd dẫn mình được ko ạ 0938880492
Nice
Check readme file
-
2 hours ago, PreviDent said:
its not working
What is not working ? Any error
-
2 hours ago, Ailee1231z said:
plz update
Fastest way to get an update is to follow this post to do DIY. Nothing difficult just running copy & paste and running a script
-
1 hour ago, Bubbnlilrock said:
i can’t get it working
What is the error ,message?
Have you use H5GG before? If not, please complete some tutorial first. -
4 hours ago, Xcxcf15 said:
does patching effect all the fileds? Steps, callbak, killeverything and force reach or there is way to hook only one field
It allows patch any number of place, but u need to specify clearly where do you want to patch
-
1 hour ago, Xcxcf15 said:
Is it possible to hook “killEverything” and make it return true?
If it possible how?
[Token(Token = "0x60010F8")]
[Address(RVA = "0x196F4E0", Offset = "0x196F4E0", VA = "0x196F4E0")]
[IteratorStateMachine(typeof(<Move_Internal>d__86))]
public IEnumerator Move_Internal(int steps, CallBack done, bool killEverything = false, bool forceReach = false)
{
return null;
}
Yes, you can hook with H5GG. But that required a patch in Binary.
-
On 6/29/2023 at 10:15 PM, bunlope said:
I greatly appreciate it. But I didn't get it. So, to get the game hacked, do I simply need to download and install the IPA hack as usual or do I also need to follow some DIY instructions?
Follow the DIY for any version update.
I may be not be able to provide update frequently.
if you can follow the DIY, you don’t need to wait for me
-
6 hours ago, Juullyan said:
“I can not log in to the game from curent region”
I got that pop up, how to fix that :((
Sorry, I have no idea about the region.
per LeePham, VPN might be required if game developer blocks you location from access. -
Modded/Hacked App: Eversoul
Bundle ID: com.kakaogames.eversoul
iTunes Store Link: https://apps.apple.com/us/app/eversoul/id1626247427
Mod Requirements:
- Non-Jailbroken/Jailed or Jailbroken iPhone/iPad/iPod Touch.
- Sideloadly / Cydia Impactor or alternatives.
- A Computer Running Windows/macOS/Linux with iTunes installed.
Hack Features:- hp (heal to full hp every 1.2 second)
- mp (heal to full mp every 1.2 second)
- one hit killNOTE:
1. Locate the little Eevee icon to open the cheat menu
2. Wait for 10-30 seconds depends on your device performance. You will see a Cheat button appear at the bottom left corner of the menu.
3. Press it and then click "Toggle Cheat"
Jailbreak required hack(s): https://iosgods.com/forum/5-game-cheats-hack-requests/
Modded Android APK(s): https://iosgods.com/forum/68-android-section/
For more fun, check out the Club(s): https://iosgods.com/clubs/
iOS Hack Download IPA Link:
Hidden Content
React or reply to this topic to see the hidden content & download link. 👀
PC Installation Instructions:
STEP 1: If necessary, uninstall the app if you have it installed on your iDevice. Some hacked IPAs will install as a duplicate app. Make sure to back it up so you don't lose your progress.
STEP 2: Download the pre-hacked .IPA file from the link above to your computer. To download from the iOSGods App, see this tutorial topic.
STEP 3: Download Sideloadly and install it on your PC.
STEP 4: Open/Run Sideloadly on your computer, connect your iOS Device, and wait until your device name shows up.
STEP 5: Once your iDevice appears, drag the modded .IPA file you downloaded and drop it inside the Sideloadly application.
STEP 6: You will now have to enter your iTunes/Apple ID email login, press "Start" & then you will be asked to enter your password. Go ahead and enter the required information.
STEP 7: Wait for Sideloadly to finish sideloading/installing the hacked IPA. If there are issues during installation, please read the note below.
STEP 8: Once the installation is complete and you see the app on your Home Screen, you will need to go to Settings -> General -> Profiles/VPN & Device Management. Once there, tap on the email you entered from step 6, and then tap on 'Trust [email protected]'.
STEP 9: Now go to your Home Screen and open the newly installed app and everything should work fine. You may need to follow further per app instructions inside the hack's popup in-game.
NOTE: iOS/iPadOS 16 and later, you must enable Developer Mode. For free Apple Developer accounts, you will need to repeat this process every 7 days. Jailbroken iDevices can also use Sideloadly/Filza/IPA Installer to normally install the IPA with AppSync. If you have any questions or problems, read our Sideloadly FAQ section of the topic and if you don't find a solution, please post your issue down below and we'll do our best to help! If the hack does work for you, post your feedback below and help out other fellow members that are encountering issues.
Credits:
- Happy Secret
- @LeePham
Cheat Video/Screenshots:N/A
-
113
-
10
-
6
-
2
-
11
-
5
-
-
Version 1.8.4:
Today is a remarkable day for H5GG enhanced menu. Here brings a monster improvement to JSPlugin. With this latest update, you can now interact directly with Unity User Object, with a bit of setup, you can use Unity User Object directly inside your JSPlugin. It is almost like a JS-binding of Unity, crazy! See below for more details:
1. Introducing Unity User Object wrapper. You can wrap ALL Unity User Object with in JS Plug-in. YES, it is pretty much like what we did earlier for UnityArray, UnityList, and UnityDictionary.
With a bit of setup like below, you can use dot (.) notation OR [] notation to directly access fields and methods!!!!!
e.g. let myPlayer = new UnityObject(<<Player Object Address>>);
myPlayer.loadFields([“score”,”exp”,”_anyfieldname”]); //You can have as many field as you like. Of course add only those you need.
myPlayer.loadMethods([“void set_exp(int32)”,”int32 get_exp()”,”void _anymethodname()”]); //You can have as many method as you like.
Then, you can do myPlayer.score=999, myPlayer.exp, ”_anyfieldname”myPlayer.set_exp(999), myPlayer.get_exp(),etc
You may find all the field names, method name from Unity dump or using my Unity Static Analyzer JSPlugin. Again, it support all Unity User Objects.
Note Currently it only support following field / param / return data type: void, int8, int16, int32, int64, single, double, boolean, text (readonly), string (readonly), for pointer please use int64.
Combining UnityObject, UnityArray, UnityList, and UnityDictionary, you can pretty much program the cheat logic with Unity User Object intuitively in JSPlugin.2. Further improved core Unity Framework to handle Enum better. YES, you can now directly see Enum value in Unity User Object Explorer.
Enum is used heavily to classify objects. It is a very important identifier for us to filter object we want to access.
3. Minor bug fix on a number of bugs.note:
1. This is a Beta release and for Unity Game only. It is good for Hack developer who want to test their limits only. Not for general user who do not familiar with Unity. For general user who do not familiar with Unity, it is still a good tool to use, but you cannot maximise its value.
2. Performance could be bad, if the game has a lot class definition. It is tradeoff for features and performance for now. If you don't like it, you may skip these in your JS plugin. It won’t affect your performance if you are not using it.v1.8.4: https://iosddl.net/880ac3724c32077c/H5GG_with_ARM_and_Unity_v1.8.4.zip
-
3
-
1
-
-
27 minutes ago, Xcxcf15 said:
I’m trying to learn by myself, I found it but nothing happen when I change it. Do you know why? Photo
In Mini Football, there are two teams of players. They called players as Stick in game. In a football match, there are 11 players in a team and 2 teams meaning 22 players. There also has two judges, also called Stick. So, there is 24 Sticks in game. Every Stick has it own PlayerAttributes. So, there are 24 PlayerAttributes objects. You need to be able to find right object to edit
Take a look at my script for Mini Football, you will be able to understand how to find the right Stick.
see my cheat shared in free non-jailbroken cheat. I have included the script there
-
14 hours ago, Xcxcf15 said:
Patching
Patching and Hooking on non-jailbroken device are standard features of H5GG.
-
4 hours ago, Xcxcf15 said:
Can Used for offsets hacking?
What do you mean by offset hacking?
H5GG Enhanced Menu Tutorial - JSPlug-in 101
in H5GG & iGameGod
Posted
if you success in creating cheat script with option 2…the. Option 3 is not far away.
if you can’t even make cheat with option 2, don’t even think about option 3. Option 3 is much advanced